How to Use Base64 Encoding for Data Security
Created on 26 September, 2024 | Converter tools | 70 views | 5 minutes read
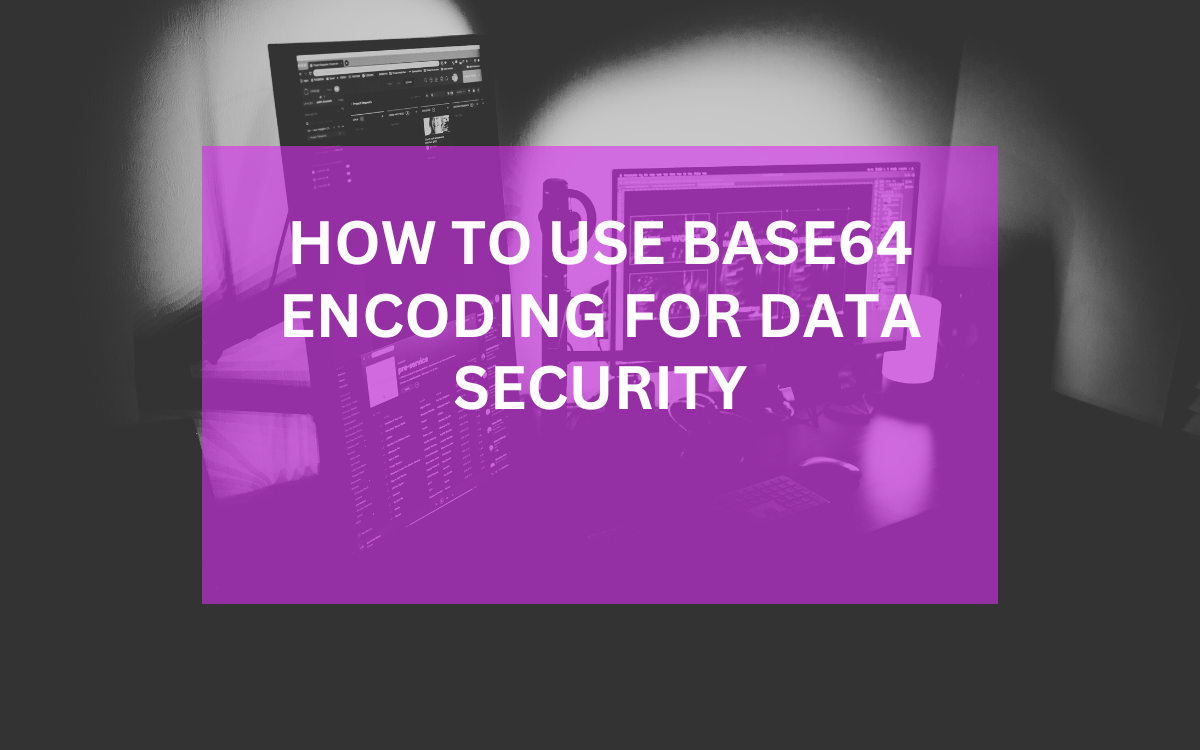
Learn how to use Base64 encoding for data security, including practical implementations, benefits, and best practices for effect
In the realm of data security, Base64 encoding plays a significant role. This technique transforms binary data into an ASCII string format, making it easier to handle and transmit. Whether you're a developer or a tech enthusiast, understanding how to use Base64 encoding can enhance your data security practices. This article delves into the various aspects of Base64 encoding, explaining its applications, benefits, and best practices.
What is Base64 Encoding?
Base64 encoding is a method for converting binary data into a text format. The process involves encoding data into a set of 64 printable characters, which makes it easier to transmit over media that only supports text. This encoding scheme is commonly used in data handling, including email transmission and data storage.
The Basics of Base64 Encoding
Base64 encoding transforms binary data into a string of text using a specific set of 64 characters. These characters include uppercase letters (A-Z), lowercase letters (a-z), digits (0-9), and two additional symbols (typically '+' and '/'). The primary purpose of this encoding is to ensure that data remains intact without modification during transport.
How It Works
When data is encoded in Base64, it is divided into groups of 6 bits each. Each 6-bit group is then mapped to a character from the Base64 alphabet. For instance, the binary sequence 001000
would be mapped to the character Q
. This method ensures that binary data can be represented in a text-only format, making it suitable for systems that don't handle raw binary data well.
For more in-depth technical details, you can refer to RFC 4648, which specifies Base64 encoding.
Why Use Base64 Encoding for Data Security?
Base64 encoding is not inherently a security measure. Instead, it serves several practical purposes that contribute to better data handling and, indirectly, security.
Compatibility and Transmission
One of the primary reasons to use Base64 encoding is compatibility. Many systems and protocols are designed to handle text data rather than binary data. For example, when sending binary files over email or embedding images in HTML, Base64 encoding ensures that the data remains intact.
Email and Web Integration
Base64 encoding is often used to encode email attachments and inline images. This encoding ensures that binary data is transmitted correctly across email systems that may not handle raw binary data well. Similarly, web developers use Base64 encoding to embed images directly into HTML or CSS files, streamlining data transfer and reducing the number of HTTP requests.
Data Integrity and Error Prevention
Another advantage of Base64 encoding is that it helps preserve data integrity. By encoding binary data into a textual format, you reduce the risk of data corruption during transmission. This is particularly important when data needs to be transferred across different systems or mediums that may not handle binary data correctly.
Implementing Base64 Encoding in Your Projects
Implementing Base64 encoding in your projects is relatively straightforward. Various programming languages provide built-in functions or libraries for encoding and decoding Base64 data. Below, we'll cover how to use Base64 encoding in several popular languages.
Base64 Encoding in Python
In Python, you can use the base64
module to handle encoding and decoding.
import base64
# Encode data
data = b'Hello, World!'
encoded_data = base64.b64encode(data)
print(encoded_data) # Output: b'SGVsbG8sIFdvcmxkIQ=='
# Decode data
decoded_data = base64.b64decode(encoded_data)
print(decoded_data) # Output: b'Hello, World!'
This simple code snippet demonstrates how to encode and decode data using Python's base64
module. The b64encode
function converts binary data into a Base64-encoded string, while b64decode
performs the reverse operation.
Base64 Encoding in JavaScript
In JavaScript, Base64 encoding can be accomplished using built-in functions.
// Encode data
let data = 'Hello, World!';
let encodedData = btoa(data);
console.log(encodedData); // Output: 'SGVsbG8sIFdvcmxkIQ=='
// Decode data
let decodedData = atob(encodedData);
console.log(decodedData); // Output: 'Hello, World!'
The btoa
function encodes a string into Base64, while atob
decodes it. Note that these functions work with strings, so you may need to handle binary data differently.
Base64 Encoding in Java
In Java, you can use the java.util.Base64
class for encoding and decoding.
import java.util.Base64;
// Encode data
String data = "Hello, World!";
String encodedData = Base64.getEncoder().encodeToString(data.getBytes());
System.out.println(encodedData); // Output: 'SGVsbG8sIFdvcmxkIQ=='
// Decode data
byte[] decodedBytes = Base64.getDecoder().decode(encodedData);
String decodedData = new String(decodedBytes);
System.out.println(decodedData); // Output: 'Hello, World!'
The Base64
class provides a convenient way to handle Base64 encoding and decoding in Java applications.
Best Practices for Using Base64 Encoding
While Base64 encoding is a useful tool, it's essential to follow best practices to ensure its effective use in data security.
Use for Non-Sensitive Data
Base64 encoding is not a security measure but a data representation format. Therefore, avoid using it to encode sensitive information. Base64 encoding does not provide encryption or obfuscation, so it should not be relied upon to secure confidential data.
Combine with Other Security Measures
For enhanced security, combine Base64 encoding with other security measures such as encryption. Encrypting data before encoding it in Base64 adds an extra layer of protection, ensuring that even if the Base64-encoded data is intercepted, it remains secure.
Monitor Encoding Overhead
Base64 encoding increases the size of the data by approximately 33%. When dealing with large datasets or bandwidth constraints, be mindful of this overhead. Evaluate whether the benefits of Base64 encoding outweigh the additional data size.
Handle Encoding Errors Gracefully
When implementing Base64 encoding, handle potential errors gracefully. Ensure that your application can manage encoding and decoding failures, such as incorrect padding or invalid characters. This approach helps maintain data integrity and avoids disruptions in your application.
Resources
For further reading and detailed information on Base64 encoding, consider the following resources:
- RFC 4648: Base64 Encoding - The official specification for Base64 encoding.
- Wikipedia: Base64 - A comprehensive overview of Base64 encoding and its uses.
- MDN Web Docs: Base64 Encoding - JavaScript documentation for Base64 encoding.
Conclusion
In summary, Base64 encoding is a valuable tool for handling binary data in text formats. It facilitates compatibility and preserves data integrity during transmission. However, it's important to remember that Base64 encoding is not a security measure by itself. For secure data handling, combine Base64 encoding with encryption and other security practices.
By understanding and applying Base64 encoding effectively, you can enhance your data management processes and ensure smoother data integration across various systems.
Popular posts
-
Top 10 AI Tools Everyone Should Know About in 2024!
AI Tools | 248 views
-
20 Free AI Tools That Are Making People Rich
AI Tools | 191 views
-
26 Best YouTube to MP3 Converting Tools For 2024!
YouTube Tools | 180 views
-
How to Prevent Domain Fraud and Protect Your Revenue
Domain Management | 173 views
-
Understanding Facial Recognition: Impacts and Ethics
AI Tools | 148 views